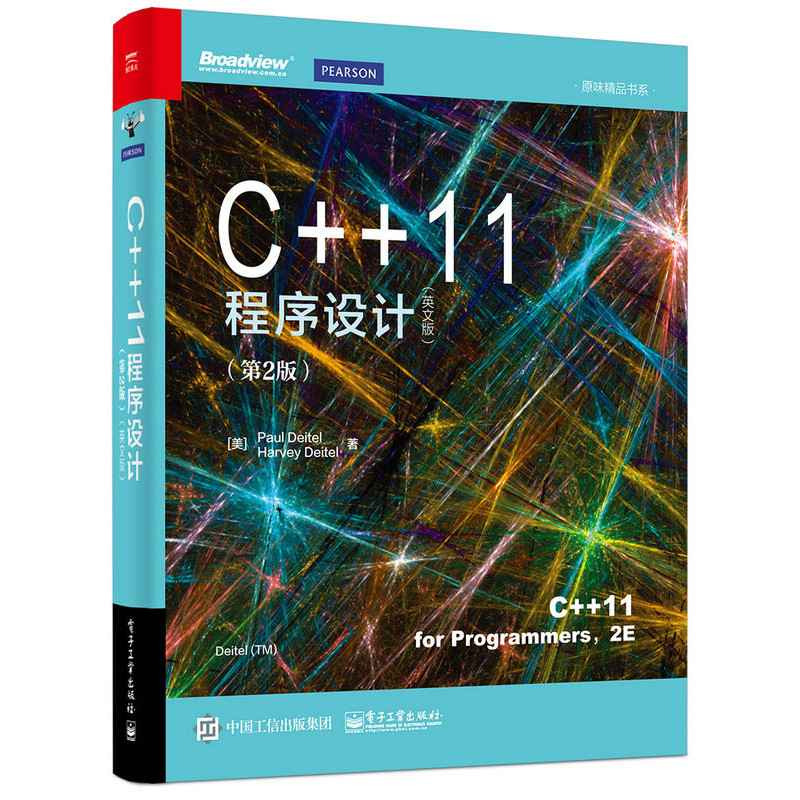
包邮C++11程序设计-(第2版)-(英文版)
1星价
¥88.8
(6.0折)
2星价¥88.8
定价¥148.0

暂无评论
图文详情
- ISBN:9787121272646
- 装帧:暂无
- 册数:暂无
- 重量:暂无
- 开本:16开
- 页数:819
- 出版时间:2016-04-01
- 条形码:9787121272646 ; 978-7-121-27264-6
内容简介
本书利用活代码深入研究 C++11 和 C++ 标准库。内容包括 C++11 的新特性,基于模板的标准库容器、迭代器和算法,C++ 标准库字符串,C++ 标准库数组,构造重要类,面向对象编程的案例研究,异常处理,等等。本书示例丰富,包含了从计算机科学、商业、模拟、游戏和其他主题中挑选出来的各种示例程序,并在三个具有工业强度的 C++11 编译器上对代码进行了测试;书中配有大量的插图,包括图表、线条图、UML 图、程序和程序输出。 本书适合具有一定高级语言编程背景的程序员阅读。
目录
目录
第24章及附录F~K为PDF文档,请访问www.informit.com/title/9780133439854或博文官网(http://www.broadview.com.cn/27264)。
前言
1 Introduction
1.1 Introduction
1.2 C++
1.3 Object Technology
1.4 Typical C++ Development Environment
1.5 Test-Driving a C++ Application
1.6 Operating Systems
1.6.1 Windows—A Proprietary Operating System
1.6.2 Linux—An Open-Source Operating System
1.6.3 Apple's OS X; Apple's iOS for iPhone , iPad and iPod Touch Devices
1.6.4 Google's Android
1.7 C++11 and the Open Source Boost Libraries
1.8 Web Resources
2 Introduction to C++ Programming, Input/Output and Operators
2.1 Introduction
2.2 First Program in C++: Printing a Line of Text
2.3 Modifying Our First C++ Program
2.4 Another C++ Program: Adding Integers
2.5 Arithmetic
2.6 Decision Making: Equality and Relational Operators
2.7 Wrap-Up
3 Introduction to Classes, Objects and Strings
3.1 Introduction
3.2 Defining a Class with a Member Function
3.3 Defining a Member Function with a Parameter
3.4 Data Members, set Member Functions and get Member Functions
3.5 Initializing Objects with Constructors
3.6 Placing a Class in a Separate File for Reusability
3.7 Separating Interface from Implementation
3.8 Validating Data with set Functions
3.9 Wrap-Up
4 Control Statements: Part 1; Assignment, ++ and -- Operators
4.1 Introduction
4.2 Control Structures
4.3 if Selection Statement
4.4 if … else Double-Selection Statement
4.5 while Repetition Statement
4.6 Counter-Controlled Repetition
4.7 Sentinel-Controlled Repetition
4.8 Nested Control Statements
4.9 Assignment Operators
4.10 Increment and Decrement Operators
4.11 Wrap-Up
5 Control Statements: Part 2; Logical Operators
5.1 Introduction
5.2 Essentials of Counter-Controlled Repetition
5.3 for Repetition Statement
5.4 Examples Using the for Statement
5.5 do … while Repetition Statement
5.6 switch Multiple-Selection Statement
5.7 break and continue Statements
5.8 Logical Operators
5.9 Confusing the Equality ( == ) and Assignment ( = ) Operators
5.10 Wrap-Up
6 Functions and an Introduction to Recursion
6.1 Introduction
6.2 Math Library Functions
6.3 Function Definitions with Multiple Parameters
6.4 Function Prototypes and Argument Coercion
6.5 C++ Standard Library Headers
6.6 Case Study: Random Number Generation
6.7 Case Study: Game of Chance; Introducing enum
6.8 C++11 Random Numbers
6.9 Storage Classes and Storage Duration
6.10 Scope Rules
6.11 Function Call Stack and Activation Records
6.12 Functions with Empty Parameter Lists
6.13 Inline Functions
6.14 References and Reference Parameters
6.15 Default Arguments
6.16 Unary Scope Resolution Operator
6.17 Function Overloading
6.18 Function Templates
6.19 Recursion
6.20 Example Using Recursion: Fibonacci Series
6.21 Recursion vs. Iteration
6.22 Wrap-Up
7 Class Templates array and vector ;
Catching Exceptions
7.1 Introduction
7.2 array s
7.3 Declaring array s
7.4 Examples Using array s
7.4.1 Declaring an array and Using a Loop to Initialize the array 's Elements
7.4.2 Initializing an array in a Declaration with an Initializer List
7.4.3 Specifying an array 's Size with a Constant Variable and Setting array Elements with Calculations
7.4.4 Summing the Elements of an array
7.4.5 Using Bar Charts to Display array Data Graphically
7.4.6 Using the Elements of an array as Counters
7.4.7 Using array s to Summarize Survey Results
7.4.8 Static Local array s and Automatic Local array s
7.5 Range-Based for Statement
7.6 Case Study: Class GradeBook Using an array to Store Grades
7.7 Sorting and Searching array s
7.8 Multidimensional array s
7.9 Case Study: Class GradeBook Using a Two-Dimensional array
7.10 Introduction to C++ Standard Library Class Template vector
7.11 Wrap-Up
8 Pointers
8.1 Introduction
8.2 Pointer Variable Declarations and Initialization
8.3 Pointer Operators
8.4 Pass-by-Reference with Pointers
8.5 Built-In Arrays
8.6 Using const with Pointers
8.6.1 Nonconstant Pointer to Nonconstant Data
8.6.2 Nonconstant Pointer to Constant Data
8.6.3 Constant Pointer to Nonconstant Data
8.6.4 Constant Pointer to Constant Data
8.7 sizeof Operator
8.8 Pointer Expressions and Pointer Arithmetic
8.9 Relationship Between Pointers and Built-In Arrays
8.10 Pointer-Based Strings
8.11 Wrap-Up
9 Classes: A Deeper Look; Throwing Exceptions
9.1 Introduction
9.2 Time Class Case Study
9.3 Class Scope and Accessing Class Members
9.4 Access Functions and Utility Functions
9.5 Time Class Case Study: Constructors with Default Arguments
9.6 Destructors
9.7 When Constructors and Destructors Are Called
9.8 Time Class Case Study: A Subtle Trap—Returning a Reference or a Pointer to a private Data Member
9.9 Default Memberwise Assignment
9.10 const Objects and const Member Functions
9.11 Composition: Objects as Members of Classes
9.12 friend Functions and friend Classes
9.13 Using the this Pointer
9.14 static Class Members
9.15 Wrap-Up
10 Operator Overloading; Class string
10.1 Introduction
10.2 Using the Overloaded Operators of Standard Library Class string
10.3 Fundamentals of Operator Overloading
10.4 Overloading Binary Operators
10.5 Overloading the Binary Stream Insertion and Stream Extraction Operators
10.6 Overloading Unary Operators
10.7 Overloading the Unary Prefix and Postfix ++ and -- Operators
10.8 Case Study: A Date Class
10.9 Dynamic Memory Management
10.10 Case Study: Array Class
10.10.1 Using the Array Class
10.10.2 Array Class Definition
10.11 Operators as Member vs. Non-Member Functions
10.12 Converting Between Types
10.13 explicit Constructors and Conversion Operators
10.14 Overloading the Function Call Operator ()
10.15 Wrap-Up
11 Object-Oriented Programming: Inheritance
11.1 Introduction
11.2 Base Classes and Derived Classes
11.3 Relationship between Base and Derived Classes
11.3.1 Creating and Using a CommissionEmployee Class
11.3.2 Creating a BasePlusCommissionEmployee Class Without Using Inheritance
11.3.3 Creating a CommissionEmployee – BasePlusCommissionEmployee Inheritance Hierarchy
11.3.4 CommissionEmployee – BasePlusCommissionEmployee Inheritance Hierarchy Using protected Data
11.3.5 CommissionEmployee – BasePlusCommissionEmployee Inheritance Hierarchy Using private Data
11.4 Constructors and Destructors in Derived Classes
11.5 public , protected and private Inheritance
11.6 Software Engineering with Inheritance
11.7 Wrap-Up
12 Object-Oriented Programming: Polymorphism
12.1 Introduction
12.2 Introduction to Polymorphism: Polymorphic Video Game
12.3 Relationships Among Objects in an Inheritance Hierarchy
12.3.1 Invoking Base-Class Functions from Derived-Class Objects
12.3.2 Aiming Derived-Class Pointers at Base-Class Objects
12.3.3 Derived-Class Member-Function Calls via Base-Class Pointers
12.3.4 Virtual Functions and Virtual Destructors
12.4 Type Fields and switch Statements
12.5 Abstract Classes and Pure virtual Functions
12.6 Case Study: Payroll System Using Polymorphism
12.6.1 Creating Abstract Base Class Employee
12.6.2 Creating Concrete Derived Class SalariedEmployee
12.6.3 Creating Concrete Derived Class CommissionEmployee
12.6.4 Creating Indirect Concrete Derived Class BasePlusCommissionEmployee
12.6.5 Demonstrating Polymorphic Processing
12.7 (Optional) Polymorphism, Virtual Functions and Dynamic Binding“Under the Hood”
12.8 Case Study: Payroll System Using Polymorphism and Runtime Type Information with Downcasting, dynamic_cast , typeid and
type_info
12.9 Wrap-Up
13 Stream Input/Output: A Deeper Look
13.1 Introduction
13.2 Streams
13.2.1 Classic Streams vs. Standard Streams
13.2.2 iostream Library Headers
13.2.3 Stream Input/Output Classes and Objects
13.3 Stream Output
13.3.1 Output of char * Variables
13.3.2 Character Output Using Member Function put
13.4 Stream Input
13.4.1 get and getline Member Functions
13.4.2 istream Member Functions peek , putback and ignore
13.4.3 Type-Safe I/O
13.5 Unformatted I/O Using read , write and gcount
13.6 Introduction to Stream Manipulators
13.6.1 Integral Stream Base: dec , oct , hex and setbase
13.6.2 Floating-Point Precision ( precision , setprecision )
13.6.3 Field Width ( width , setw )
13.6.4 User-Defined Output Stream Manipulators
13.7 Stream Format States and Stream Manipulators
13.7.1 Trailing Zeros and Decimal Points ( showpoint )
13.7.2 Justification ( left , right and internal )
13.7.3 Padding ( fill , setfill )
13.7.4 Integral Stream Base ( dec , oct , hex , showbase )
13.7.5 Floating-Point Numbers; Scientific and Fixed Notation( scientific , fixed )
13.7.6 Uppercase/Lowercase Control ( uppercase )
13.7.7 Specifying Boolean Format ( boolalpha )
13.7.8 Setting and Resetting the Format State via Member Function flags
13.8 Stream Error States
13.9 Tying an Output Stream to an Input Stream
13.10 Wrap-Up
14 File Processing
14.1 Introduction
14.2 Files and Streams
14.3 Creating a Sequential File
14.4 Reading Data from a Sequential File
14.5 Updating Sequential Files
14.6 Random-Access Files
14.7 Creating a Random-Access File
14.8 Writing Data Randomly to a Random-Access File
14.9 Reading from a Random-Access File Sequentially
14.10 Case Study: A Transaction-Processing Program
14.11 Object Serialization
14.12 Wrap-Up
15 Standard Library Containers and Iterators
15.1 Introduction
15.2 Introduction to Containers
15.3 Introduction to Iterators
15.4 Introduction to Algorithms
15.5 Sequence Containers
15.5.1 vector Sequence Container
15.5.2 list Sequence Container
15.5.3 deque Sequence Container
15.6 Associative Containers
15.6.1 multiset Associative Container
15.6.2 set Associative Container
15.6.3 multimap Associative Container
15.6.4 map Associative Container
15.7 Container Adapters
15.7.1 stack Adapter
15.7.2 queue Adapter
15.7.3 priority_queue Adapter
15.8 Class bitset
15.9 Wrap-Up
16 Standard Library Algorithms
16.1 Introduction
16.2 Minimum Iterator Requirements
16.3 Algorithms
16.3.1 fill , fill_n , generate and generate_n
16.3.2 equal , mismatch and lexicographical_compare
16.3.3 remove , remove_if , remove_copy and remove_copy_if
16.3.4 replace , replace_if , replace_copy and replace_copy_if
16.3.5 Mathematical Algorithms
16.3.6 Basic Searching and Sorting Algorithms
16.3.7 swap , iter_swap and swap_ranges
16.3.8 copy_backward , merge , unique and reverse
16.3.9 inplace_merge , unique_copy and reverse_copy
16.3.10 Set Operations
16.3.11 lower_bound , upper_bound and equal_range
16.3.12 Heapsort
16.3.13 min , max , minmax and minmax_element
16.4 Function Objects
16.5 Lambda Expressions
16.6 Standard Library Algorithm Summary
16.7 Wrap-Up
17 Exception Handling: A Deeper Look
17.1 Introduction
17.2 Example: Handling an Attempt to Divide by Zero
17.3 Rethrowing an Exception
17.4 Stack Unwinding
17.5 When to Use Exception Handling
17.6 Constructors, Destructors and Exception Handling
17.7 Exceptions and Inheritance
17.8 Processing new Failures
17.9 Class unique_ptr and Dynamic Memory Allocation
17.10 Standard Library Exception Hierarchy
17.11 Wrap-Up
18 Introduction to Custom Templates
18.1 Introduction
18.2 Class Templates
18.3 Function Template to Manipulate a Class-Template Specialization Object
18.4 Nontype Parameters
18.5 Default Arguments for Template Type Parameters
18.6 Overloading Function Templates
18.7 Wrap-Up
19 Class string and String Stream Processing: A Deeper Look
19.1 Introduction
19.2 string Assignment and Concatenation
19.3 Comparing string s
19.4 Substrings
19.5 Swapping string s
19.6 string Characteristics
19.7 Finding Substrings and Characters in a string
19.8 Replacing Characters in a string
19.9 Inserting Characters into a string
19.10 Conversion to Pointer-Based char * Strings
19.11 Iterators
19.12 String Stream Processing
19.13 C++11 Numeric Conversion Functions
19.14 Wrap-Up
20 Bits, Characters, C Strings and struct s
20.1 Introduction
20.2 Structure Definitions
20.3 typedef
20.4 Example: Card Shuffling and Dealing Simulation
20.5 Bitwise Operators
20.6 Bit Fields
20.7 Character-Handling Library
20.8 C String-Manipulation Functions
20.9 C String-Conversion Functions
20.10 Search Functions of the C String-Handling Library
20.11 Memory Functions of the C String-Handling Library
20.12 Wrap-Up
21 Other Topics
21.1 Introduction
21.2 const_cast Operator
21.3 mutable Class Members
21.4 namespace s
21.5 Operator Keywords
21.6 Pointers to Class Members ( .* and ->* )
21.7 Multiple Inheritance
21.8 Multiple Inheritance and virtual Base Classes
21.9 Wrap-Up
22 ATM Case Study, Part 1:Object-Oriented Design with the UML
22.1 Introduction
22.2 Introduction to Object-Oriented Analysis and Design
22.3 Examining the ATM Requirements Document
22.4 Identifying the Classes in the ATM Requirements Document
22.5 Identifying Class Attributes
22.6 Identifying Objects' States and Activities
22.7 Identifying Class Operations
22.8 Indicating Collaboration Among Objects
22.9 Wrap-Up
23 ATM Case Study, Part 2:Implementing an Object-Oriented Design
23.1 Introduction
23.2 Starting to Program the Classes of the ATM System
23.3 Incorporating Inheritance into the ATM System
23.4 ATM Case Study Implementation
23.4.1 Class ATM
23.4.2 Class Screen
23.4.3 Class Keypad
23.4.4 Class CashDispenser
23.4.5 Class DepositSlot
23.4.6 Class Account
23.4.7 Class BankDatabase
23.4.8 Class Transaction
23.4.9 Class BalanceInquiry
23.4.10 Class Withdrawal
23.4.11 Class Deposit
23.4.12 Test Program ATMCaseStudy.cpp
23.5 Wrap-Up
A Operator Precedence and Associativity
B ASCII Character Set
C Fundamental Types
D Number Systems
D.1 Introduction
D.2 Abbreviating Binary Numbers as Octal and Hexadecimal Numbers
D.3 Converting Octal and Hexadecimal Numbers to Binary Numbers
D.4 Converting from Binary, Octal or Hexadecimal to Decimal
D.5 Converting from Decimal to Binary, Octal or Hexadecimal
D.6 Negative Binary Numbers: Two's Complement Notation
E Preprocessor
E.1 Introduction
E.2 #include Preprocessing Directive
E.3 #define Preprocessing Directive: Symbolic Constants
E.4 #define Preprocessing Directive: Macros
E.5 Conditional Compilation
E.6 #error and #pragma Preprocessing Directives
E.7 Operators # and ##
E.8 Predefined Symbolic Constants
E.9 Assertions
E.10 Wrap-Up
Index
线上章节及附录
第 24 章及附录 F~K 为 PDF 文档,请访问 www.informit.com/title/9780133439854
或博文官网( http://www.broadview.com.cn/27264 )。
展开全部
本类五星书
浏览历史
本类畅销
-
硅谷之火-人与计算机的未来
¥13.7¥39.8 -
造神:人工智能神话的起源和破除 (精装)
¥32.7¥88.0 -
超简单:用python+ ChatGPT让excel飞起来
¥48.4¥79.0 -
专业导演教你拍好短视频
¥13.8¥39.9 -
数学之美
¥41.0¥69.0 -
系统性创新手册(管理版)
¥42.6¥119.0 -
软件工程(第2版)
¥14.3¥39.0 -
计算机网络技术
¥24.1¥33.0 -
.NET安全攻防指南(下册)
¥89.0¥129.0 -
.NET安全攻防指南(上册)
¥89.0¥129.0 -
人工智能的底层逻辑
¥55.3¥79.0 -
数据挖掘技术与应用
¥52.0¥75.0 -
SOLIDWORKS中文版实用教程
¥134.9¥149.9 -
PYTHON机器学习:基础、算法与实战
¥71.3¥99.0 -
计算
¥92.2¥128.0 -
MIDJOURNEY AI绘画从入门到精通
¥71.5¥98.0 -
商业产品分析:从用户数据获得商业洞见的数据科学方法
¥89.0¥129.0 -
老年人学电脑
¥34.9¥49.9 -
人工智能AI摄影与后期修图从小白到高手:MIDJOURNEY+PHOTOSHOP
¥56.9¥98.0 -
人月神话(纪念典藏版)
¥68.6¥98.0